TOGA Topic 2: Game Logic
- miketfkee
- Oct 30, 2020
- 2 min read
Updated: Nov 21, 2020
Week 2 of TOGA taught us how to use conditional statements (if, else, while, boolean, switch/case). These function in a similar way to other coding languages like Python and Java, and hence were a nice refresher on the topic, especially the switch/case.
The worksheet walked us through how each conditional statement worked (making note of the edge cases - wherein the code could go wrong if you forgot to do >= or <= and just entered < / >). Then, it asked to mix the if and else statements by making 3 conditions that needed to be fulfilled.
int number;
cout << ("Enter a number.");
cin >> number;
if (number < 7)
{
cout << ("Lower than 7");
}
else if (number == 7)
{
cout << ("It is 7");
}
else
{
cout << ("Higher than 7");
}
This code took in a number that the user inputted and saved it to the variable "number". It then checked if the number was smaller, equal or bigger than 7, which covered all edge cases.
The last worksheet task combined all previously explained conditionals into one task:
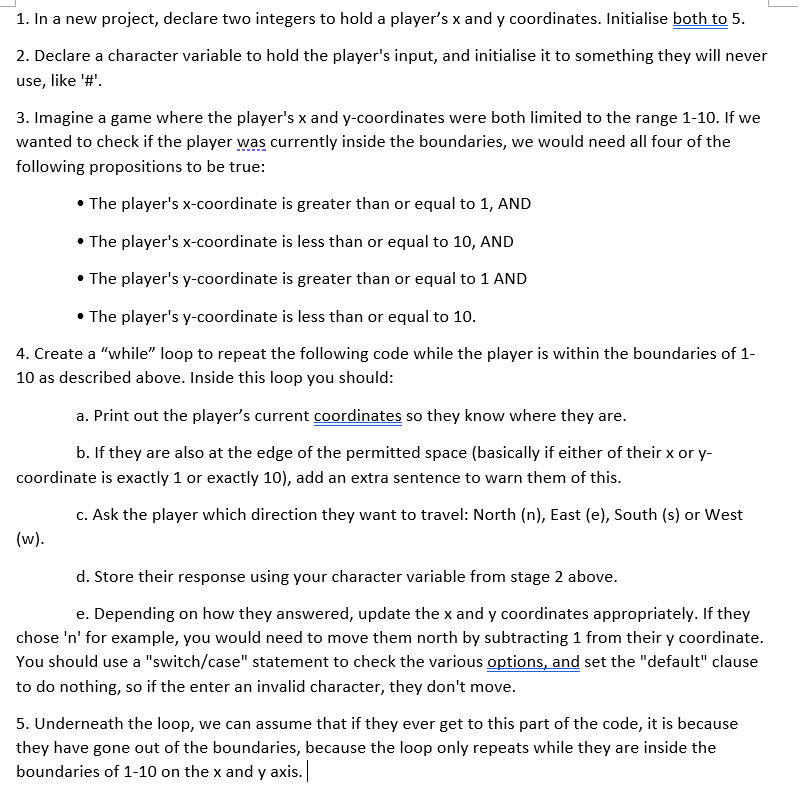
My solution is as follows:
int main()
{
int xCoord = 5;
int yCoord = 5;
char direction = '#';
while (xCoord >= 1 && xCoord <= 10 && yCoord >= 1 && yCoord <= 10)
{
cout << "Your X-coordinate is " << xCoord << " and your Y-coordinate is " << yCoord << ".\n";
if (xCoord == 10 || yCoord == 10 || xCoord == 1 || yCoord == 1)
{
cout << "You are too close to the border!\n";
}
cout << "Which direction do you want to travel? n/e/s/w \n";
cin >> direction;
switch (direction)
{
case 'n':
yCoord++;
break;
case 'e':
xCoord++;
break;
case 's':
yCoord--;
break;
case 'w':
xCoord--;
break;
default:
cout << "Invalid input.\n";
}
}
cout << "You left the safe zone.\n";
}
Here I utilize switch/case as it is more efficient than writing multiple if/else statements. While the player is within the "safe zone", the code will repeatedly ask the user for a correct input (n/e/s/w). Any inputs that are not accepted will be treated as default and result in "Invalid Input". If the user enters an accepted input, the code will add or subtract from the xCoord or yCoord respectively. If the user's coordinates are on the border, an extra line is printed notifying the user of this.
Comments