TOGA Week 4: Loops and Arrays
- miketfkee
- Nov 24, 2020
- 2 min read
Updated: Dec 2, 2020
This workshop will get you using "for" loops and multidimensional arrays in your code, so you can minimize the burden of repetitive tasks, especially when working with classes.
Having previously used for loops in my code for multiple projects, including the single-button game and the idle clicker, I was relatively familiar with how to use loops. However, I had not used multidimensional arrays much and so was fairly new to the concept.
The first step required us to create a for loop. This would usually be done with the following code:
for (int i = 0; i < x; i++)
wherein x is the number of times you want to run the loop for. Each time the code runs, the integer i is increased by 1, and so on until it becomes the same number as x. In the workshop's case, it wanted us to run the code 5 times, and therefore x = 5.
Next, the workshop asked for the loop to be reversed. For this, the for loop needed to be modified like so:
for (int i = 5; i > x; i--)
Now the code starts from 5 and subtracts 1 each time the loop is run.
Lastly, the workshop asks for:
Modify the loop so it starts at 1, and then doubles the number each time until it hits a number greater than 1500.
For this, only the for loop needs to be changed once again:
for (int i = 1; i <= 1500; i=i*2)
{
cout << (i) << endl;
}
Next, I worked on Step 3 of the worksheet.
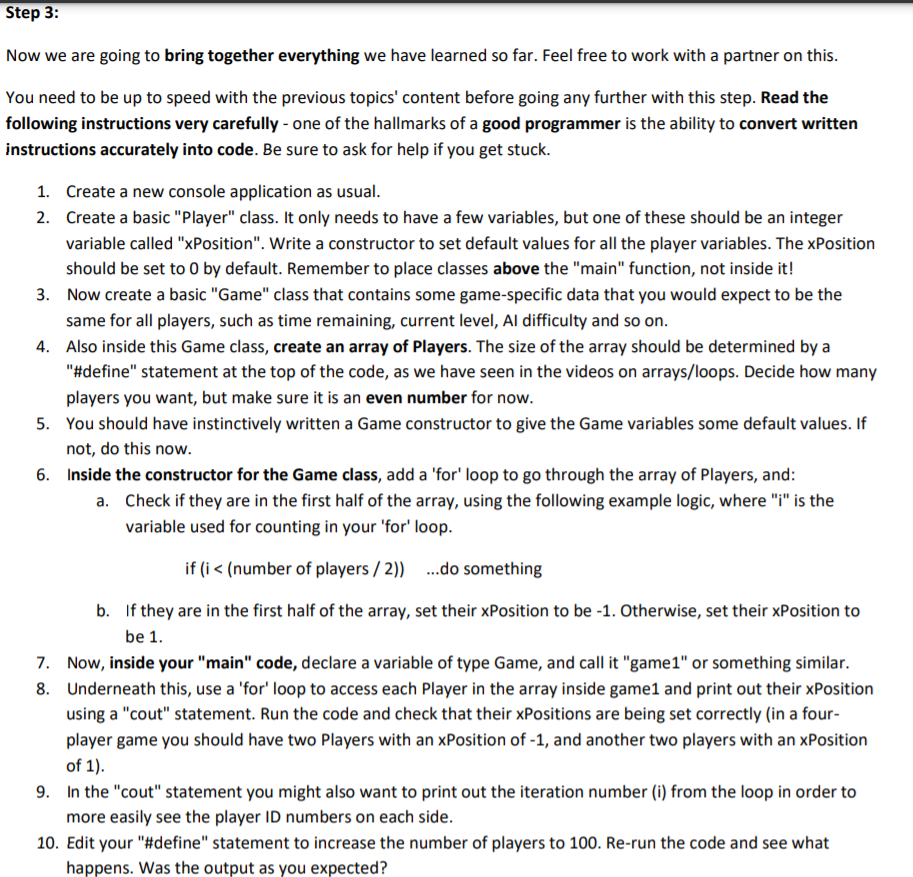
My code for this step was as follows:
#include <string>
#include <iostream>
#define NUM_OF_PLAYERS 6
using namespace std;
class Player
{
public:
int xPosition;
string playerName;
Player()
{
xPosition = 0;
playerName = "default";
}
};
class Game
{
public:
double timeRemaining;
int currentLevel;
int botLevel;
Player numberOfPlayers[NUM_OF_PLAYERS];
Game()
{
timeRemaining = 120.0;
currentLevel = 1;
botLevel = 1;
for (int i = 0; i < 6; i++)
{
if (i < (NUM_OF_PLAYERS / 2))
{
(numberOfPlayers[i].xPosition = -1);
}
else
{
(numberOfPlayers[i].xPosition = 1);
}
}
}
};
int main()
{
Game game1;
for (int i = 0; i < NUM_OF_PLAYERS; i++)
{
cout << (game1.numberOfPlayers[i].xPosition) << endl;
}
}
I set up NUM_OF_PLAYERS to represent the number 6, firstly. Then I created two classes, Player and Game. As required by the steps, the Player class had the X Position defaulted to 0 in the constructor. Next, the Game class had an array, numberOfPlayers, with NUM_OF_PLAYERS inside (meaning 6 players in the array). The for loop also has a if statement inside, allowing the for loop to cycle through each player in the array.
for (int i = 0; i < 6; i++)
{
if (i < (NUM_OF_PLAYERS / 2))
{
(numberOfPlayers[i].xPosition = -1);
}
else
{
(numberOfPlayers[i].xPosition = 1);
}
}
This bit of code checks the position of the players in the array. If they are in the first half of the array (in this case since there are 6 players in the array the first 3) then their X position is set to -1; elsewise it is set to 1. It then prints out the list of players' X position.
Comments